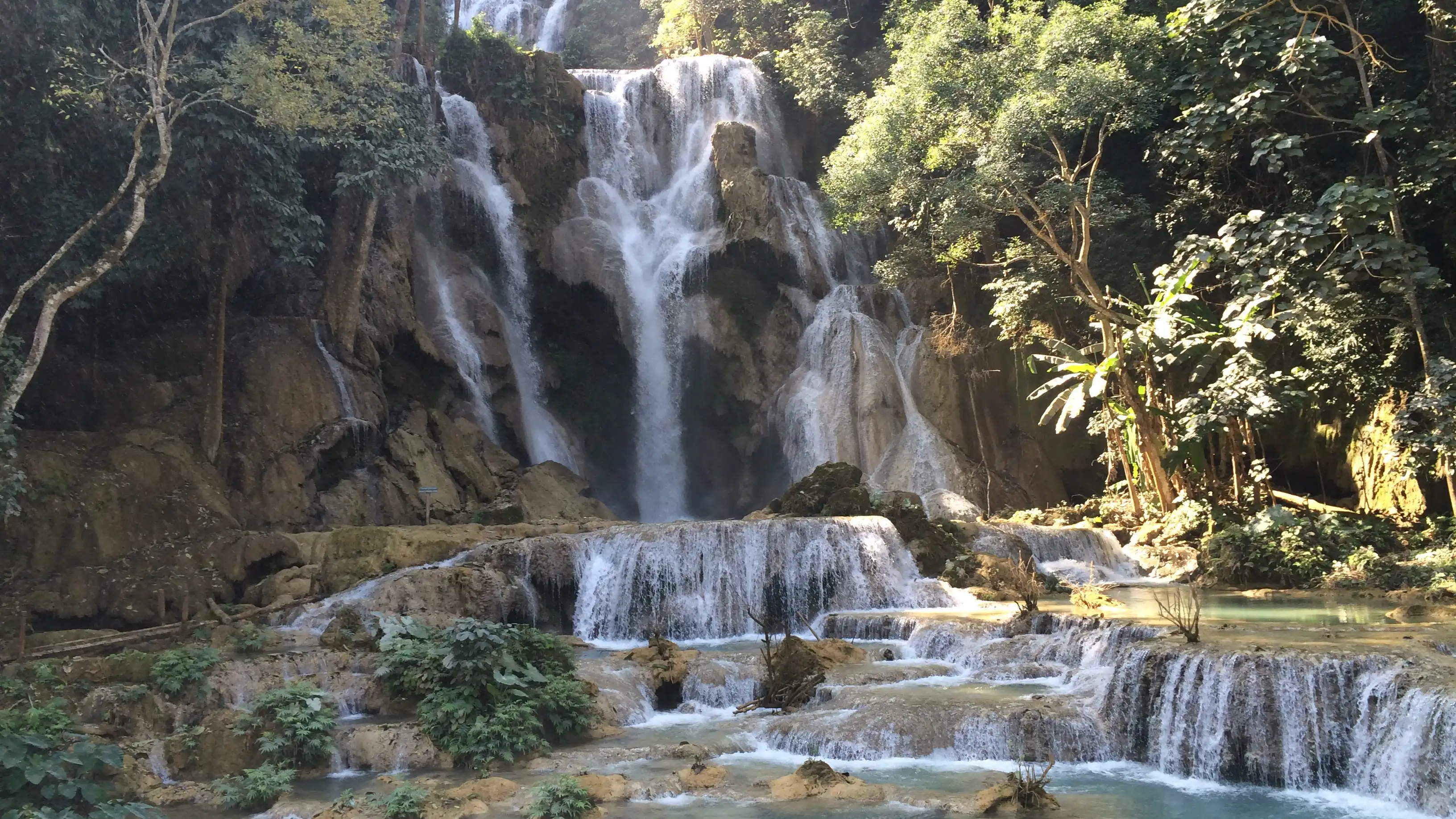
Luang Prabang, Laos (2015)
Django + Vue Experiments: Vuetify
How to use Vuetify in Django templates.
One of the advantages of adding Vue to Django is the ability to use other people’s open-sourced Vue Components right in your Django templates. This also includes the ability to use components from modern UX libraries like Vuetify.
This article will show you how to get started with using Vuetify components to enhance the UX of your Django templates. But the lessons can be applied to any 3rd-party Vue library.
Table of Contents
- Setup Vuetify
- Experiment 2a: Default Django Messages
- Experiment 2b: Vuetified Django Messages
- What’s next?
Setup Vuetify
In this case, I don’t want to create an entire Vuetify application, I just want to use bits of pieces of it in my current Django app. So I’ll follow the instructions here for adding Vuetify to an existing project.
Following the instructions from Part 1, I’m using Vite and django-vite to manage my JavaScript dependencies. I can install Vuetify with npm (npm i vuetify
) and then import it into any JavaScript file that lives in my vite config’s root
directory (/django_vue_experiments/vite_assets
).
Instantiating Vuetify in your Django template’s Vue app works the same as any other Vue app. All of the components
that you specify when you createVuetify
will be available to use within your Django template.
Here’s an example Vue app that enables Vuetify’s VAlert
component to be used in your linked Django template:
const vuetify = createVuetify({ components: { VAlert, }, icons: { defaultSet: "mdi", aliases, sets: { mdi, }, }, ssr: true,});
const RootComponent = defineComponent({ delimiters: ["[[", "]]"],});
const app = createApp(RootComponent);app.use(vuetify).mount("#vue-experiment-2b");
Let’s see what a simple Django page looks like before we use any Vuetify components.
Experiment 2a: Default Django Messages
Django’s messaging framework is useful, but very bare-bones. Creating a nice UX around message notifications will require some amount of JavaScript development — unless you use a framework like Vuetify which provides pre-built components for you.
Here’s what Django messages look like out-of-the-box, without any JavaScript.
Now let’s look at an example that uses Vuetify’s VAlert
component.
Experiment 2b: Vuetified Django Messages
My messages look a lot nicer! And I didn’t even have to do any JavaScript development to get them implemented!
This was accomplished by plugging in Vuetify’s <v-alert>
component inside of my Django template header’s {% for message in messages %}
for-loop. You can use Vue components the same way you’d use any other html component in a template.
What’s next?
Parts 1 and 2 of this series introduced a couple of very simple examples of using Vue in Django templates. But these simple — practically stateless — examples are probably not what you have in mind if you’re contemplating using Vue in Django templates.
How can we integrate Vue components into Django forms? That’s the real test — using Vue in highly interactive forms that are still managed by Django form classes.
That’s what we’ll be looking at in the next part of this series. In the meantime, you can see my work-in-progress form examples at supergood-reads before the next article gets published.
🔆